PyTorch is a powerful framework for building deep learning models. It is easy to use and integrates well with Python. This blog will explain the key features of PyTorch and show you how to use it for your own deep learning projects.
Here are the key features of PyTorch:
Dynamic computational graph: This allows you to build models that are more flexible and adaptable.
Intuitive API: The API is easy to use and understand, even for beginners.
Seamless integration with Python: PyTorch works seamlessly with Python, which makes it easy to use with other libraries and tools
What is PyTorch? PyTorch is an open-source machine learning library based on the Torch library, primarily developed for applications in deep learning and neural networks. Unlike other frameworks with static computational graphs, PyTorch uses dynamic computation, allowing for easy debugging, interactive development, and dynamic model architectures. This unique feature, known as "define-by-run," has won the hearts of researchers and developers worldwide, making PyTorch a preferred choice for both academia and industry.
Key Features of PyTorch:
Dynamic Computational Graph: PyTorch's dynamic computation graph enables seamless model development, where operations are defined as they are executed, facilitating easier debugging and more intuitive code.
Tensors and Automatic Differentiation: PyTorch offers powerful tensor operations similar to NumPy, while also providing automatic differentiation to compute gradients, a crucial aspect for training deep learning models.
GPU Acceleration: PyTorch seamlessly supports GPUs, allowing users to take advantage of the massive parallel processing capabilities and accelerating model training.
TorchScript: PyTorch's TorchScript provides a Just-In-Time (JIT) compiler, enabling users to serialize models and run them in environments where Python is not available, such as mobile devices and web browsers.
Neural Network Module: PyTorch provides a high-level module called torch.nn, simplifying the process of building complex neural network architectures.
Pythonic Interface: With a Pythonic API, PyTorch offers a familiar and intuitive development experience for Python developers, making it easy to adopt and use.
Steps to Master PyTorch : Let's dive into the steps to use PyTorch for building and training deep learning models:
Step 1: Install PyTorch Start by installing PyTorch using the appropriate installation command based on your system configuration and the desired version. For example, you can use pip to install the CPU version of PyTorch:
bashCopy code
pip install torch torchvision
Step 2: Import PyTorch Import the PyTorch library into your Python script or notebook:
pythonCopy code
import torch
import torch.nn as nn
import torch.optim as optim
Step 3: Create the Dataset Prepare your data and split it into training and testing sets. You can use PyTorch's Dataset and DataLoader classes to handle data efficiently.
pythonCopy code
from torch.utils.data import Dataset, DataLoader
class CustomDataset(Dataset):
# Implement your custom dataset here
train_dataset = CustomDataset(training_data)
train_loader = DataLoader(train_dataset, batch_size=batch_size, shuffle=True)
Step 4: Define the Model Create your neural network model by subclassing torch.nn.Module and defining the forward pass.
pythonCopy code
class MyModel(nn.Module):
def __init__(self, input_size, output_size):
super(MyModel, self).__init__()
self.fc1 = nn.Linear(input_size, 128)
self.fc2 = nn.Linear(128, output_size)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
model = MyModel(input_size, output_size)
Step 5: Define Loss Function and Optimizer Choose an appropriate loss function and optimizer for your task.
pythonCopy code
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
Step 6: Training the Model Train your model by iterating over the data batches and updating the model parameters through backpropagation.
pythonCopy code
for epoch in range(num_epochs):
for inputs, labels in train_loader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
Step 7: Evaluation Evaluate your model's performance on the test dataset.
pythonCopy code
with torch.no_grad():
correct = 0
total = 0for inputs, labels in test_loader:
outputs = model(inputs)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
accuracy = 100 * correct / total
print(f"Test Accuracy: {accuracy}%")
PyTorch has revolutionized the world of deep learning with its dynamic computation graph, intuitive API, and Pythonic interface, offering developers and researchers a powerful tool for building sophisticated machine learning models. Its ease of use, GPU acceleration, and strong community support have made it a favorite choice among AI enthusiasts and industry professionals alike.
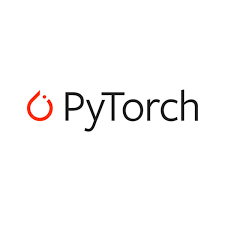
In this blog, we explored the key features of PyTorch and provided a step-by-step guide to using PyTorch for building and training deep learning models. Whether you're a seasoned data scientist or an aspiring AI enthusiast, PyTorch welcomes you to unlock the vast potential of deep learning and create cutting-edge AI solutions that shape the future of technology. Happy coding!
Comments